Defending from Vulnerabilities: Endless Redirection Loop in URL Parameters
If the redirections are improperly handled, they often lead to open redirection attacks. However, if the number of redirection attempts is not implemented, it can cause a redirection loop, leading to an application-level denial of service and resource exhaustion attack.
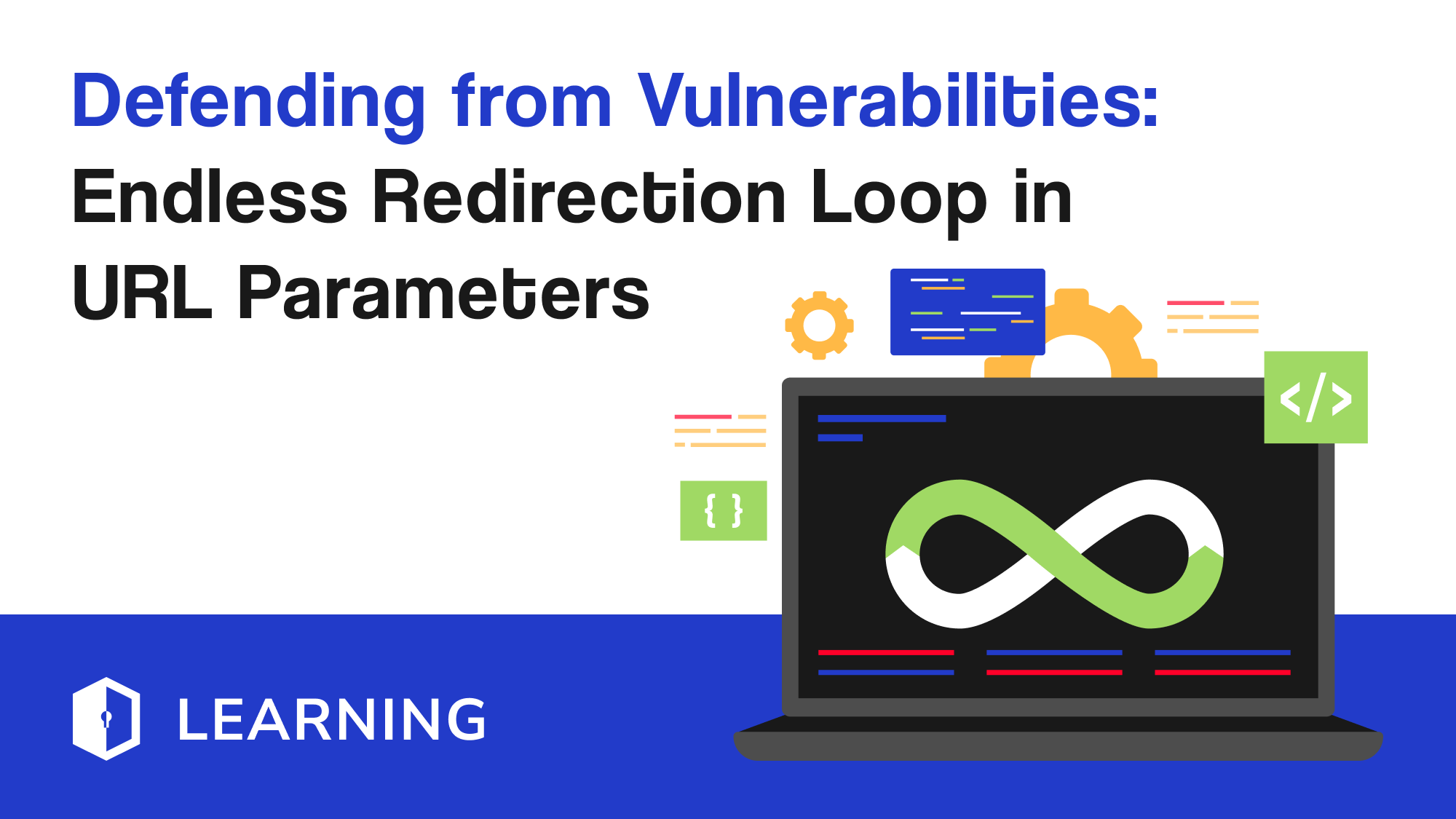
If the redirections are improperly handled, they often lead to open redirection attacks. However, if the number of redirection attempts is not implemented, it can cause a redirection loop, leading to an application-level denial of service and resource exhaustion attack.
In this week's series, we explore a vulnerability where an application uses a return_url=
parameter (or any similar) on the login screen, such as <application_host>/login/?return_url=/myprofile
.
While this implementation is not vulnerable to open redirection or DOM XSS, an attacker can supply a URL such as <application_host>/login/?return_url=https://blog.defensiumlabs.com
. Instead of redirecting to a valid or invalid page, this results in an endless redirection loop, causing resource exhaustion and an application-level denial of service (DoS).
This scenario highlights the need to validate and handle redirect URLs to prevent such attacks properly.
Understanding the Attack
- Return URL Parameter:
- The application uses a
return_url
parameter to redirect users after login. - The parameter is not properly validated, allowing arbitrary URLs.
- The application uses a
- Attack Vector:
- An attacker supplies a malicious URL that causes the application to enter an endless redirection loop.
- This loop leads to resource exhaustion and a denial of service.
Defensive Strategy
To defend against this specific redirection loop scenario, follow these steps:
Validate the Return URL Parameter: Ensure that the return_url
parameter points to a valid, trusted URL within the application and rejects any external URLs or malformed paths.
Javascript Example:
const express = require('express');
const url = require('url');
const app = express();
const isValidReturnUrl = (returnUrl) => {
const parsedUrl = url.parse(returnUrl);
return parsedUrl.protocol === null && parsedUrl.hostname === null; // Ensure it's a relative URL
};
app.get('/login', (req, res) => {
const { return_url } = req.query;
if (return_url && !isValidReturnUrl(return_url)) {
return res.status(400).send('Invalid return URL');
}
// Proceed with login logic
// Redirect to return_url after successful login
res.redirect(return_url || '/default');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Set a Maximum Number of Redirections: Implement a mechanism to track and limit the number of allowed redirections to prevent infinite loops.
Javascript Example:
const express = require('express');
const session = require('express-session');
const url = require('url');
const app = express();
app.use(session({
secret: 'your_secret_key',
resave: false,
saveUninitialized: true
}));
const MAX_REDIRECTIONS = 5;
const isValidReturnUrl = (returnUrl) => {
const parsedUrl = url.parse(returnUrl);
return parsedUrl.protocol === null && parsedUrl.hostname === null; // Ensure it's a relative URL
};
app.get('/login', (req, res) => {
const { return_url } = req.query;
req.session.redirectCount = (req.session.redirectCount || 0) + 1;
if (req.session.redirectCount > MAX_REDIRECTIONS) {
return res.status(400).send('Too many redirects');
}
if (return_url && !isValidReturnUrl(return_url)) {
return res.status(400).send('Invalid return URL');
}
// Proceed with login logic
// Reset the redirect count after successful login
req.session.redirectCount = 0;
res.redirect(return_url || '/default');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Use a Whitelist for Allowed Redirects: Maintain a whitelist of allowed redirect URLs to ensure only trusted paths are used.
Javascript Example:
const express = require('express');
const app = express();
const ALLOWED_URLS = ['/myprofile', '/dashboard', '/settings'];
app.get('/login', (req, res) => {
const { return_url } = req.query;
if (return_url && !ALLOWED_URLS.includes(return_url)) {
return res.status(400).send('Invalid return URL');
}
// Proceed with login logic
res.redirect(return_url || '/default');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Implement Logging and Monitoring: Enable logging and monitoring to detect and respond to abnormal redirection patterns.
Javascript Example:
const express = require('express');
const winston = require('winston');
const app = express();
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.File({ filename: 'combined.log' }),
],
});
app.use((req, res, next) => {
logger.info(`${req.method} ${req.url}`);
next();
});
app.get('/login', (req, res) => {
const { return_url } = req.query;
// Log the return_url for monitoring
logger.info(`Return URL: ${return_url}`);
if (return_url && !isValidReturnUrl(return_url)) {
return res.status(400).send('Invalid return URL');
}
// Proceed with login logic
res.redirect(return_url || '/default');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Conclusion
By validating the return_url
parameter, setting a maximum number of redirections, using a whitelist for allowed redirects, and implementing logging and monitoring, you can effectively mitigate the risk of endless redirection loops in your application.
This detailed approach helps developers and security engineers understand and apply these protections in their specific scenarios.
Stay tuned for more specific attack scenarios and defensive strategies in our weekly series.