Defending from Vulnerabilities: Exploiting Object Injection for Remote Code Execution (RCE)
Object injection occurs when user-supplied input is deserialized into an object within the application without proper validation or sanitization.
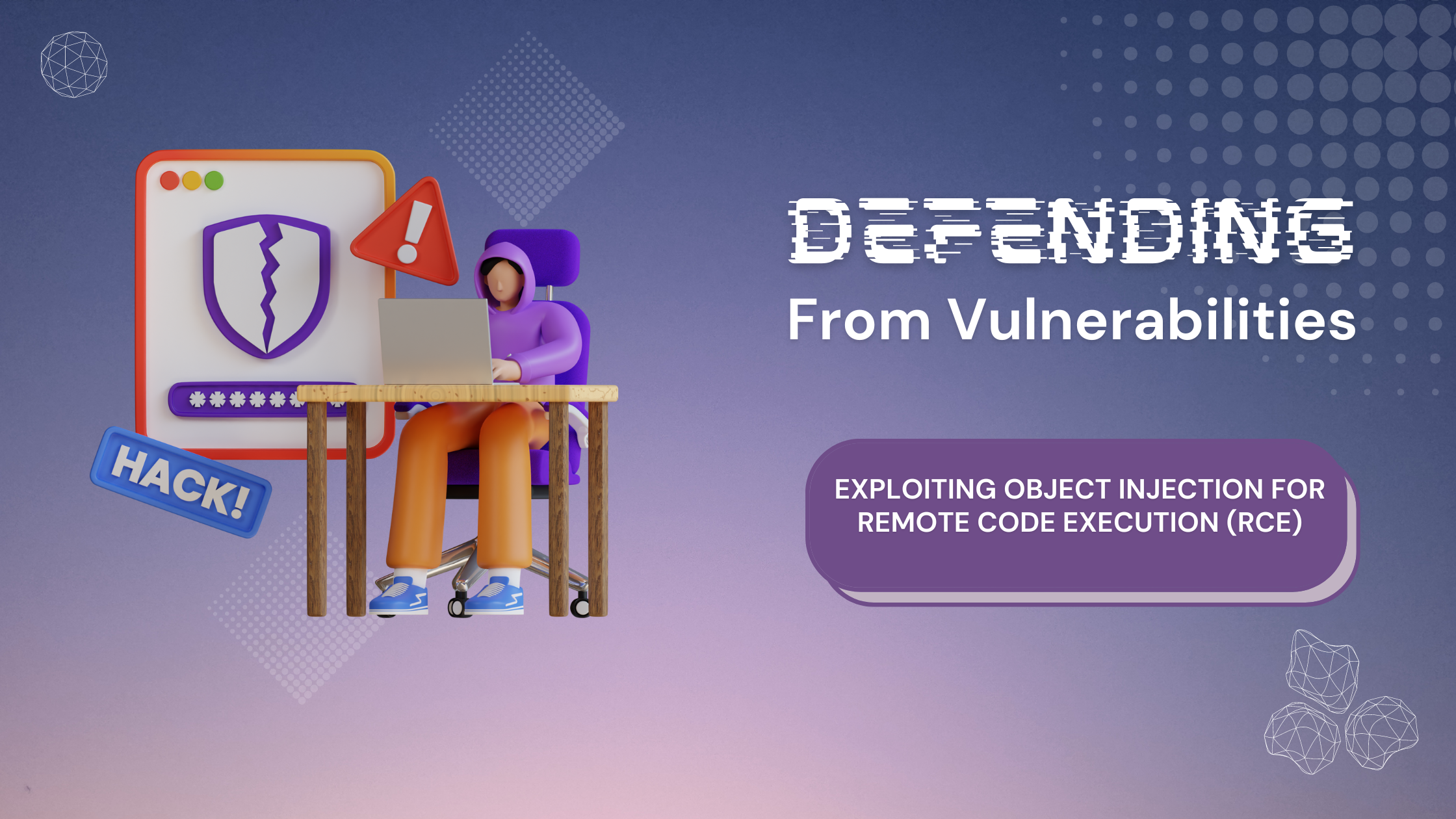
In this week's series, we delve into a critical vulnerability known as Object Injection, which can lead to severe consequences such as Remote Code Execution (RCE). Object injection occurs when user-supplied input is deserialized into an object within the application without proper validation or sanitization.
Attackers can exploit this vulnerability by manipulating serialized data to inject malicious objects into the system, leading to the execution of arbitrary code. This scenario highlights the importance of securely handling serialized data and understanding the risks associated with deserialization.
Understanding the Attack
- Object Deserialization:
- The application accepts serialized data from the user and deserializes it into an object. Deserialization involves converting the serialized data back into its original object form.
- Attack Vector:
- The attacker sends malicious serialized data to the application, which gets deserialized into an object. The object contains references to dangerous methods or properties that can trigger unwanted actions.
- This allows the attacker to execute arbitrary code or gain unauthorized access to sensitive parts of the application.
- Consequences:
- If successfully exploited, object injection can lead to Remote Code Execution (RCE), privilege escalation, or access to sensitive information.
Defensive Strategy
To defend against object injection attacks, follow these steps:
Avoid Untrusted Data Deserialization: Do not deserialize user-supplied data unless absolutely necessary. If deserialization is required, use safe and restricted formats like JSON that limit the risks associated with complex object structures.
Node.js Example using JSON instead of Object Deserialization:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.post('/process', (req, res) => {
// Validate and process the user-supplied JSON data
const data = req.body;
if (!data || typeof data !== 'object') {
return res.status(400).send('Invalid data');
}
// Process the input safely
res.send(`Data processed: ${JSON.stringify(data)}`);
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Use Safe Deserialization Libraries: Use libraries and frameworks that offer secure deserialization mechanisms. Avoid using insecure methods or libraries that allow arbitrary object deserialization.
Java Example using Safe Deserialization with Jackson:
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.DeserializationFeature;
public class SafeDeserializationExample {
public static void main(String[] args) throws Exception {
ObjectMapper mapper = new ObjectMapper();
// Disable the default typing which could lead to security risks
mapper.disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
// Simulating safe deserialization
String jsonData = "{\"name\": \"John\", \"age\": 30}";
User user = mapper.readValue(jsonData, User.class);
System.out.println("User name: " + user.getName());
}
}
class User {
private String name;
private int age;
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
Validate and Sanitize Input Data: Ensure all serialized data is validated before deserialization. Implement strong input validation to prevent malicious data from being processed.
PHP Example using Strict Input Validation for Serialized Data:
<?php
$data = $_POST['serialized_data'] ?? null;
if (!$data) {
die('No data provided');
}
// Validate the serialized data to ensure itβs safe before deserializing
if (!is_string($data) || !preg_match('/^[a-zA-Z0-9:{};"]+$/', $data)) {
die('Invalid serialized data');
}
$deserializedData = unserialize($data);
// Only proceed if the deserialized data is an expected type
if (!is_array($deserializedData)) {
die('Invalid object type');
}
// Process the deserialized data safely
print_r($deserializedData);
?>
Implement Application-Level Sandbox or Whitelisting: Implement whitelisting or sandboxing techniques to ensure that only trusted classes or types are allowed during deserialization.
Python Example using Restricted Deserialization in PyYAML:
import yaml
class SafeLoader(yaml.SafeLoader):
def construct_undefined(self, node):
raise yaml.YAMLError("Unsafe deserialization detected!")
yaml.SafeLoader.add_constructor(None, SafeLoader.construct_undefined)
def safe_deserialize(yaml_data):
try:
# Use SafeLoader to avoid unsafe object deserialization
return yaml.load(yaml_data, Loader=SafeLoader)
except yaml.YAMLError as e:
return f"Deserialization error: {e}"
yaml_data = """
name: John Doe
age: 30
"""
deserialized_data = safe_deserialize(yaml_data)
print(deserialized_data)
Conclusion
By avoiding untrusted data deserialization, using safe deserialization libraries, validating and sanitizing input, and implementing whitelisting mechanisms, you can effectively defend against object injection attacks that lead to remote code execution and other critical exploits. This approach ensures that your application is robust against deserialization vulnerabilities. Stay tuned for more specific attack scenarios and defensive strategies in our weekly series.