Defending from Vulnerabilities: Persistent XSS via Lack of Internal Application Data Validation
As a developer, you must perform input validation at each stage even if the data is already stored in the application because avoiding this could still lead to a malicious input to trigger on later interaction (second degree), causing issues like cross-site scripting (XSS).
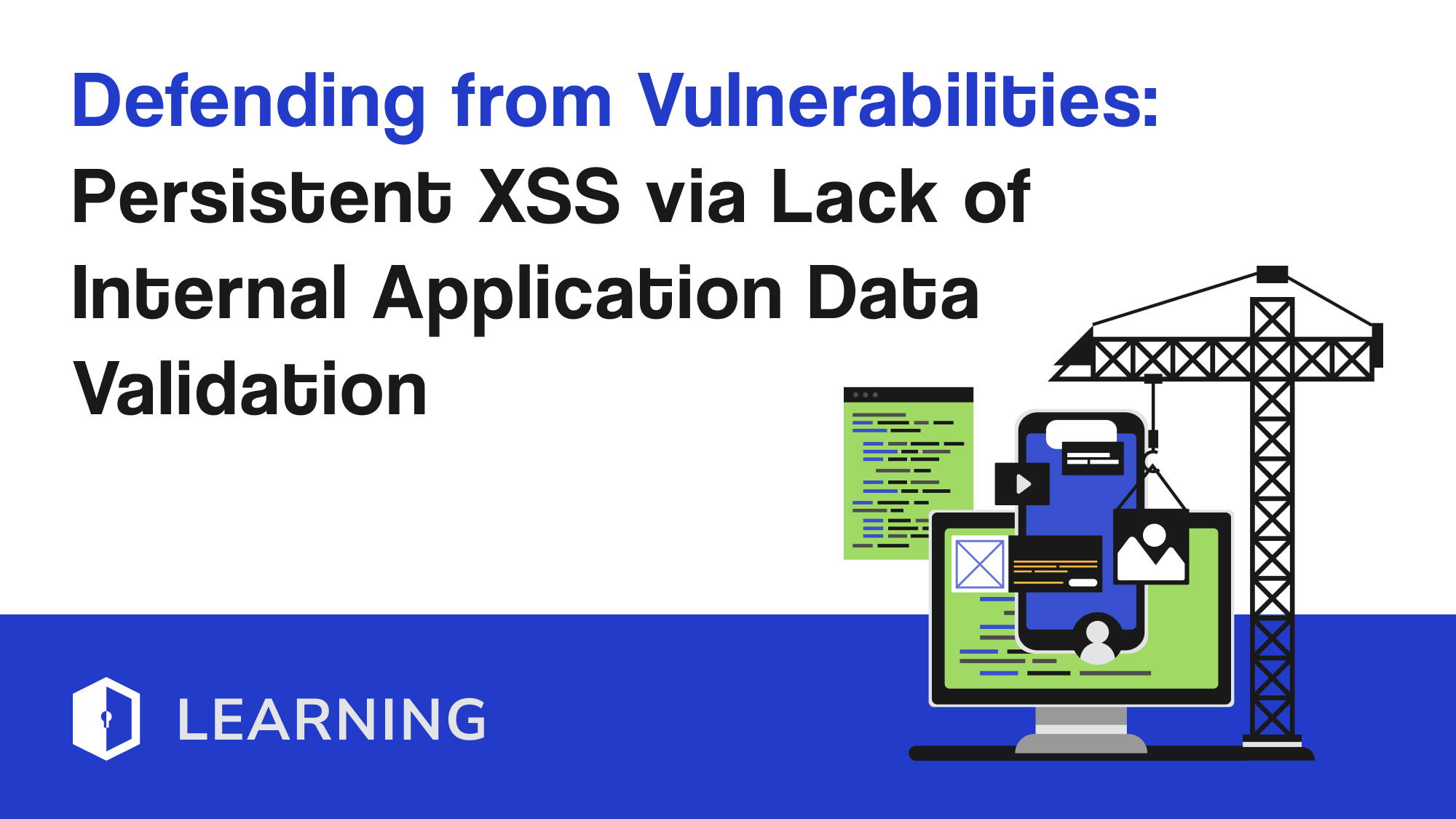
As a developer, you must perform input validation at each stage even if the data is already stored in the application because avoiding this could still lead to a malicious input to trigger on later interaction (second degree), causing issues like cross-site scripting (XSS).
In this week's series, we will explore a vulnerability where an application properly validates user-supplied input to prevent XSS attacks when a user creates a post. However, an attacker managed to insert an XSS payload that was triggered when the input was used in another feature of the application. This was due to a lack of validation for existing entries coming from the application itself, which were treated as trusted input. This scenario highlights the importance of validating input at every stage, even if it originates from within the application.
Understanding the Attack
- Input Validation on User Posts: The application validates user-supplied input to safely display it when a user creates a post, restricting XSS attacks.
- Attack Vector:
- An attacker includes an XSS payload in a user post.
- The payload is stored in the database.
- When the stored input is used in another part of the application without re-validation, the XSS payload is executed, compromising the application.
Defensive Strategy
To defend against this specific persistent XSS scenario, follow these steps:
Validate Input at Every Stage: Always validate input, even if it is coming from another part of the application and treat all data as untrusted, regardless of its origin.
Javascript Example:
const express = require('express');
const bodyParser = require('body-parser');
const validator = require('validator');
const app = express();
app.use(bodyParser.urlencoded({ extended: false }));
app.post('/create-post', (req, res) => {
let { content } = req.body;
// Validate and sanitize user input
if (!validator.isLength(content, { min: 1, max: 500 })) {
return res.status(400).send('Content length must be between 1 and 500 characters.');
}
content = validator.escape(content);
// Save the sanitized content to the database
// db.savePost(content);
res.send('Post created successfully');
});
app.get('/display-post/:id', async (req, res) => {
const postId = req.params.id;
// Fetch the post from the database
const post = await db.getPostById(postId);
// Re-validate and sanitize the content before using it
if (!post || !validator.isLength(post.content, { min: 1, max: 500 })) {
return res.status(400).send('Invalid post content.');
}
const safeContent = validator.escape(post.content);
res.send(`<div>${safeContent}</div>`);
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Use Output Encoding: Apply proper output encoding to all data before rendering it in the browser to prevent XSS.
Javascript Example:
app.get('/display-post/:id', async (req, res) => {
const postId = req.params.id;
// Fetch the post from the database
const post = await db.getPostById(postId);
// Encode the content for safe HTML output
const safeContent = validator.escape(post.content);
res.send(`<div>${safeContent}</div>`);
});
Sanitize Data on Storage and Retrieval: Sanitize data both when it is stored and when it is retrieved to ensure no malicious scripts are executed.
Javascript Example:
// Sanitize data before storing
const sanitizedContent = validator.escape(content);
db.savePost(sanitizedContent);
// Sanitize data when retrieving
const post = await db.getPostById(postId);
const safeContent = validator.escape(post.content);
res.send(`<div>${safeContent}</div>`);
Implement Content Security Policies (CSP): Use CSP to mitigate the risk of XSS attacks by restricting the sources of executable scripts.
Javascript Example:
const helmet = require('helmet');
app.use(helmet.contentSecurityPolicy({
directives: {
defaultSrc: ["'self'"],
scriptSrc: ["'self'"],
objectSrc: ["'none'"],
upgradeInsecureRequests: [],
}
}));
By validating input at every stage, using output encoding, sanitizing data on storage and retrieval, and implementing Content Security Policies, you can effectively mitigate the risk of persistent XSS attacks in your application.
This detailed approach helps developers and security engineers understand and apply these protections in their specific scenarios.
Stay tuned for more specific attack scenarios and defensive strategies in our weekly series.