Defending from Vulnerabilities: Server-Side Template Injection (SSTI)
SSTI can lead to severe outcomes, including remote code execution (RCE), data theft, and privilege escalation. This scenario underscores the importance of adequately handling user input, especially when using templating engines to generate content dynamically.
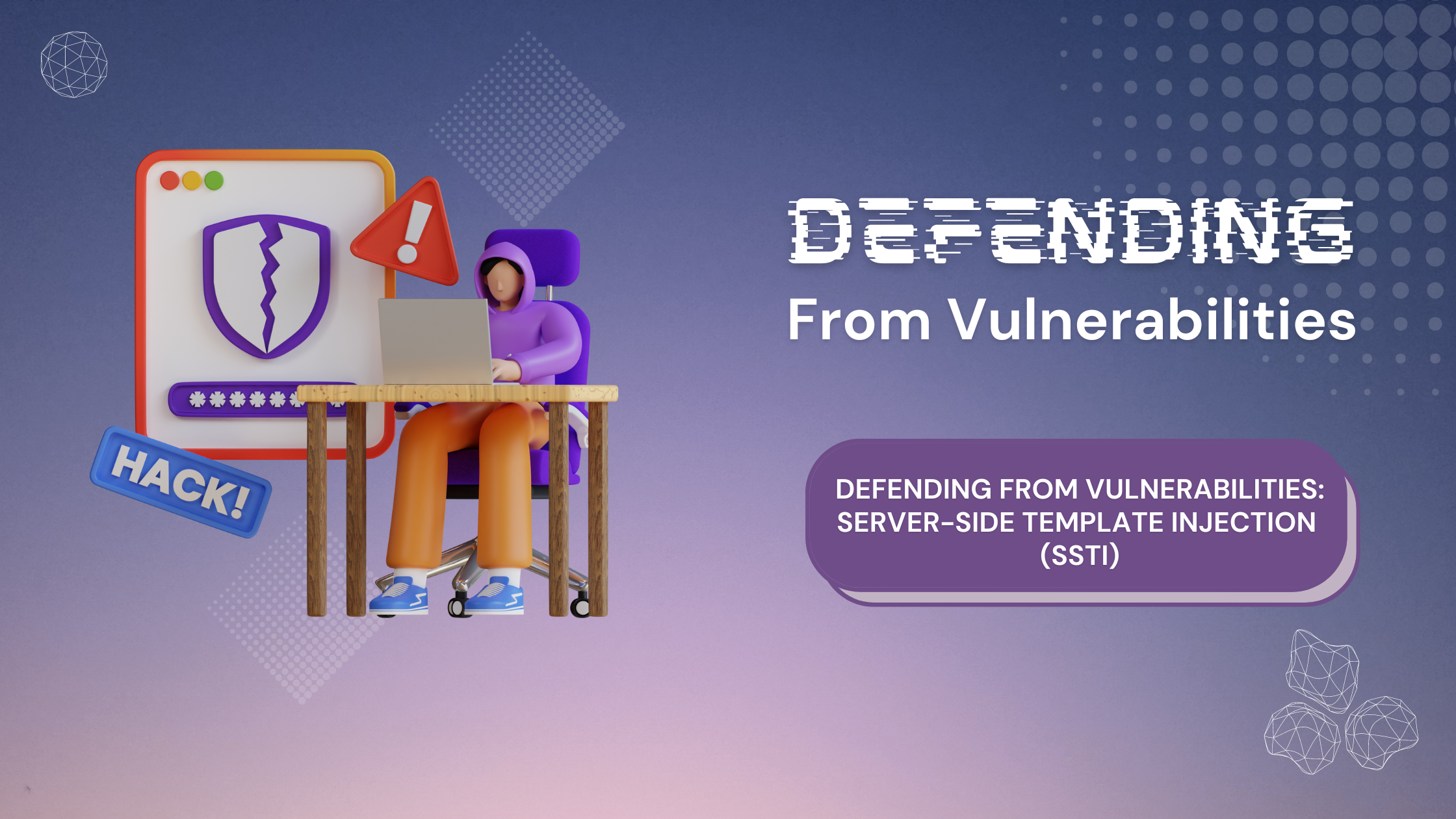
This week's series examines a server-side vulnerability known as Server-Side Template Injection (SSTI). This occurs when an attacker can inject malicious payloads into server-side templates, which are then rendered and executed by the server. SSTI can lead to severe outcomes, including remote code execution (RCE), data theft, and privilege escalation. This scenario underscores the importance of adequately handling user input, especially when using templating engines to generate content dynamically.
Understanding the Attack
- Server-Side Template Rendering:
- The application uses a server-side templating engine (e.g., Jinja2 for Python, Twig for PHP, or Pug for Node.js) to render dynamic content based on user input.
- Attack Vector:
- The attacker identifies an input field or parameter passed directly to the template without proper sanitization.
- The attacker injects a malicious payload into the input, which is then processed by the templating engine.
- Depending on the engine and the context, this could lead to remote code execution, unauthorized access to server resources, or data exfiltration.
Defensive Strategy
To defend against SSTI, follow these steps:
Avoid Directly Passing User Input to Templates: Never pass raw user input directly to templates. Instead, use context-specific escaping and filtering to ensure that any input is sanitized before being processed by the template engine.
Example:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.set('view engine', 'pug');
app.use(bodyParser.urlencoded({ extended: true }));
app.post('/greet', (req, res) => {
const { name } = req.body;
// Sanitize the user input before passing it to the template
const safeName = escapeHtml(name);
// Pass the sanitized input to the template
res.render('greet', { name: safeName });
});
function escapeHtml(unsafe) {
return unsafe
.replace(/&/g, "&")
.replace(/</g, "<")
.replace(/>/g, ">")
.replace(/"/g, """)
.replace(/'/g, "'");
}
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Use Safe Templating Functions: Utilize the templating engineβs safe rendering functions to limit the scope of what can be executed within a template. Disable or restrict the use of dangerous functions or expressions.
Example:
from flask import Flask, render_template, request, escape
app = Flask(__name__)
@app.route('/greet', methods=['POST'])
def greet():
name = request.form['name']
# Escape user input before passing to the template
safe_name = escape(name)
return render_template('greet.html', name=safe_name)
if __name__ == '__main__':
app.run(debug=True)
Implement Input Validation and Sanitization: Validate and sanitize all user inputs before they are used in the application. This includes inputs that might not be directly used in templates but could be manipulated by attackers to influence template rendering.
Example:
from django.shortcuts import render
from django.utils.html import escape
def greet(request):
if request.method == 'POST':
name = request.POST.get('name', '')
# Sanitize the user input
safe_name = escape(name)
return render(request, 'greet.html', {'name': safe_name})
Regularly Update and Patch Templating Engines: Ensure your templating engine and related dependencies are regularly updated to mitigate known vulnerabilities.
Conclusion
By avoiding direct user input in templates, using safe templating functions, implementing rigorous input validation and sanitization, and keeping templating engines up-to-date, you can effectively defend against SSTI vulnerabilities. This approach ensures that your application is robust against attacks that exploit server-side templating weaknesses. Stay tuned for more specific attack scenarios and defensive strategies in our weekly series.