Defending from Vulnerabilities: Unauthorized File Upload via Undocumented PUT Method
Publishing the undocumented APIs in an externally facing environment could allow attackers to find and use the undocumented method maliciously.
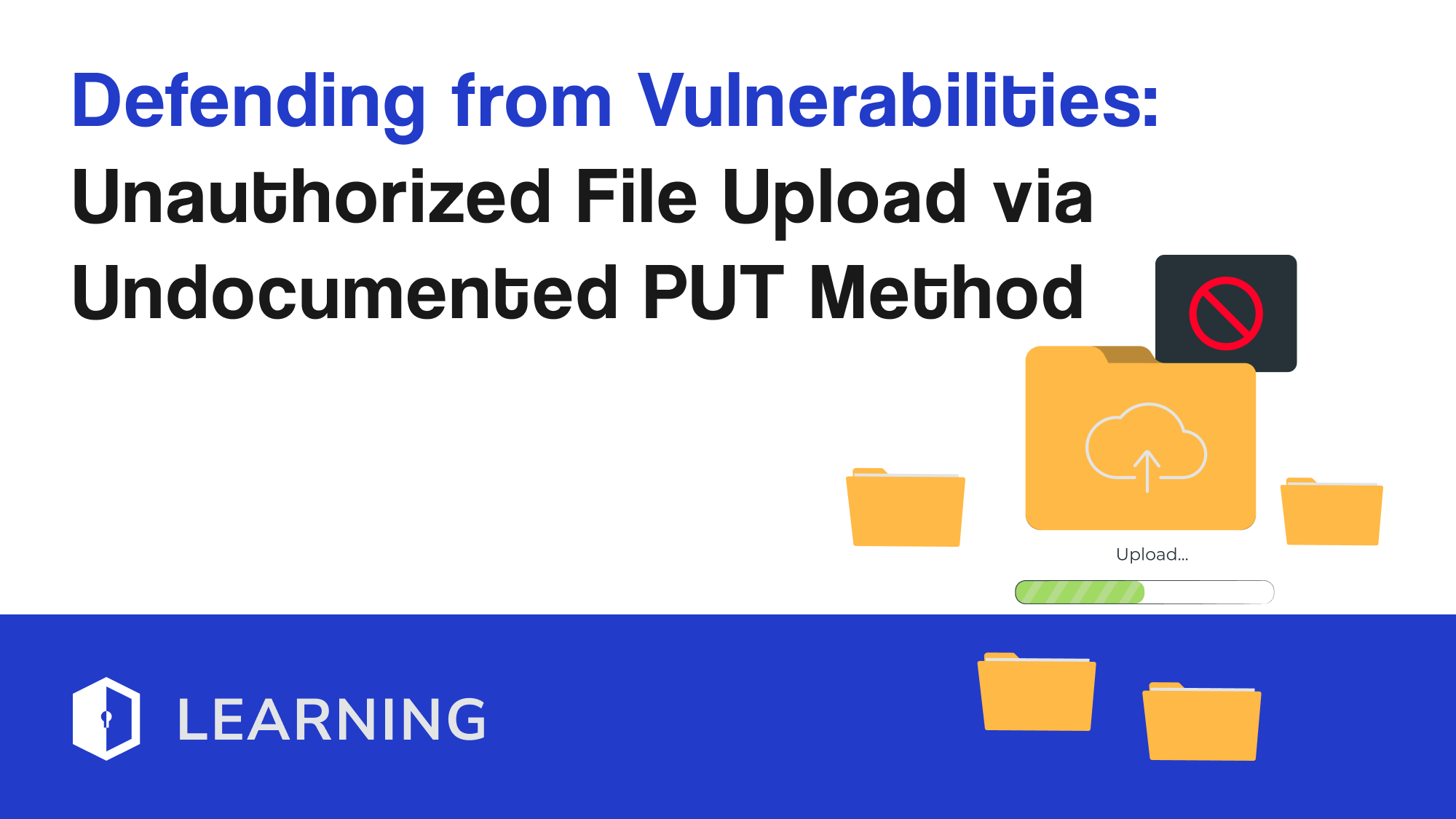
Often, developers create additional endpoints for their own debugging purposes while creating the APIs during the development process, which is never intended to be used in production. However, publishing the undocumented APIs in an externally facing environment could allow attackers to find and use the undocumented method maliciously.
In this week's series, we explore a vulnerability where an undocumented PUT method allows attackers to upload malicious files on the server by abusing the PUT request on the /
(root) endpoint. This scenario highlights the importance of securing undocumented endpoints and ensuring proper validation and access control for file uploads.
Understanding the Attack
- Undocumented PUT Method:
- The application has an undocumented PUT method that allows file uploads to the root endpoint (
/
).
- The application has an undocumented PUT method that allows file uploads to the root endpoint (
- Attack Vector:
- The attacker discovers and abuses the PUT method to upload malicious files to the server.
- Due to the lack of validation and access control, the server accepts and stores the malicious files.
Defensive Strategy
To defend against this specific unauthorized file upload scenario, follow these steps:
Remove or Secure Undocumented Endpoints: Ensure that all undocumented or unused endpoints are either removed or properly secured with access controls.
JavaScript Example:
First, review and remove any undocumented endpoints if they are not required:
const express = require('express');
const app = express();
// Remove the undocumented PUT endpoint if not needed
// app.put('/', async (req, res) => {
// // Logic to handle file upload
// });
app.listen(3000, () => {
console.log('Server running on port 3000');
});
If the PUT endpoint is needed internally, secure it with proper access controls:
const express = require('express');
const { isAuthenticated, isAdmin } = require('./authMiddleware'); // Middleware for authentication and admin check
const app = express();
app.put('/', isAuthenticated, isAdmin, async (req, res) => {
// Logic to handle file upload
res.send('File uploaded successfully');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Implement File Validation and Sanitization: Validate and sanitize files before accepting and storing them on the server to prevent malicious uploads.
JavaScript Example with Multer for File Uploads:
const express = require('express');
const multer = require('multer');
const { isAuthenticated, isAdmin } = require('./authMiddleware'); // Middleware for authentication and admin check
const app = express();
const upload = multer({
dest: 'uploads/',
fileFilter: (req, file, cb) => {
// Validate file type (e.g., only allow images)
if (!file.mimetype.startsWith('image/')) {
return cb(new Error('Only image files are allowed!'), false);
}
cb(null, true);
},
limits: {
fileSize: 5 * 1024 * 1024, // Limit file size to 5MB
},
});
app.put('/', isAuthenticated, isAdmin, upload.single('file'), async (req, res) => {
// Additional file sanitization logic if needed
res.send('File uploaded successfully');
});
app.use((err, req, res, next) => {
if (err instanceof multer.MulterError) {
return res.status(400).send(err.message);
} else if (err) {
return res.status(400).send(err.message);
}
next();
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Use Content Security Policies (CSP): Implement CSP to restrict the types of files that can be uploaded and served by the application.
JavaScript Example with Helmet:
const helmet = require('helmet');
app.use(helmet.contentSecurityPolicy({
directives: {
defaultSrc: ["'self'"],
scriptSrc: ["'self'"],
imgSrc: ["'self'", "data:"], // Allow images from self and data URIs
objectSrc: ["'none'"],
upgradeInsecureRequests: [],
}
}));
Enable Logging and Monitoring: Enable logging and monitoring to detect and respond to unauthorized file upload attempts.
JavaScript Logging Example with Winston:
const express = require('express');
const winston = require('winston');
const { isAuthenticated, isAdmin } = require('./authMiddleware'); // Middleware for authentication and admin check
const multer = require('multer');
const app = express();
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.File({ filename: 'combined.log' }),
],
});
const upload = multer({
dest: 'uploads/',
fileFilter: (req, file, cb) => {
// Validate file type (e.g., only allow images)
if (!file.mimetype.startsWith('image/')) {
return cb(new Error('Only image files are allowed!'), false);
}
cb(null, true);
},
limits: {
fileSize: 5 * 1024 * 1024, // Limit file size to 5MB
},
});
app.use((req, res, next) => {
logger.info(`${req.method} ${req.url}`);
next();
});
app.put('/', isAuthenticated, isAdmin, upload.single('file'), async (req, res) => {
res.send('File uploaded successfully');
});
app.use((err, req, res, next) => {
if (err instanceof multer.MulterError) {
return res.status(400).send(err.message);
} else if (err) {
return res.status(400).send(err.message);
}
next();
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Conclusion
By removing or securing undocumented endpoints, implementing file validation and sanitization, using content security policies, and enabling logging and monitoring, you can effectively mitigate the risk of unauthorized file uploads in your application.
This detailed approach helps developers and security engineers understand and apply these protections in their specific scenarios.
Stay tuned for more specific attack scenarios and defensive strategies in our weekly series.